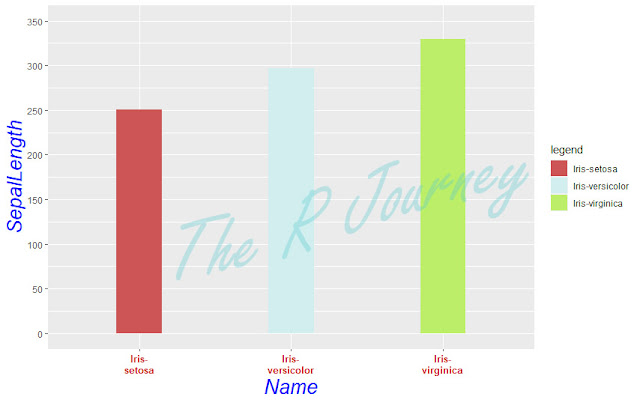
One of the strong points of R is plotting. It is possible to make highly presentable plots with the help of ggplot2 library. There are many plots available in ggplot2. The aim of this tutorial series more on configuring the different aspects of a plot, like background, grid, axis, title, border and so on. We will se how to make a simple plot and make it into nice looking plot.
The libraries and data set needed.
Library: ggplot2, stringr
Dataset: iris (to be loaded from local drive)
IDE: RStudio
# First load the library
library(ggplot2)
# The set your working directory
setwd("C:/R_Train") # please replace with your own directory
# Read the file and create a data frame
tbl <- read.csv("Sample Data/iris.csv")
Get the csv file from here.
data source
For this tutorial Bar chart is used to illustrate the modifications/enhancements you can do.
Base plot
Base plot
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity")
From the documentation “ Sometimes, bar charts are used not as a distributional summary, but instead of a dotplot. Generally, it's preferable to use a dotplot (see geom_point) as it has a better data-ink ratio. However, if you do want to create this type of plot, you can set y to the value you have calculated, and use stat='identity' ”
Output
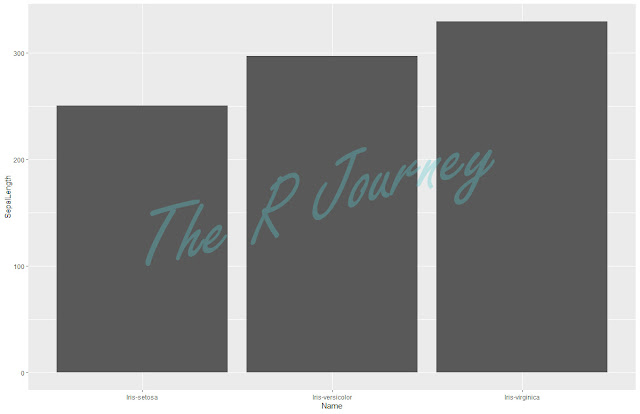
The graph is basic and nothing fancy about it. Let’s configure or improve the appearance.
Let’s start with x-axis. First modify the Axis title.
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20))
Output
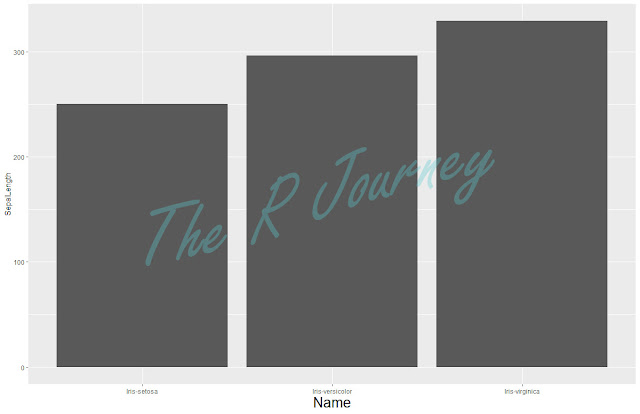
Code
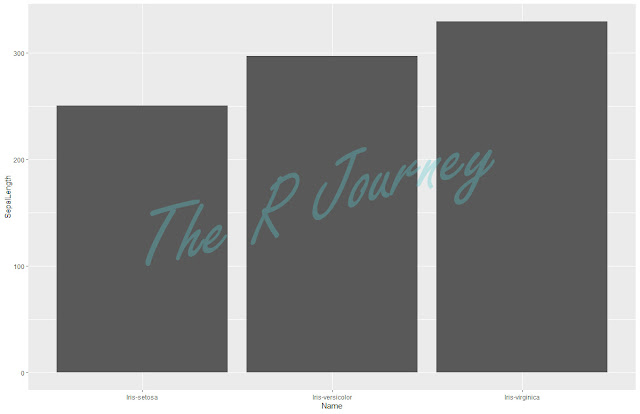
The graph is basic and nothing fancy about it. Let’s configure or improve the appearance.
Let’s start with x-axis. First modify the Axis title.
- Increase the Font size
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20))
Output
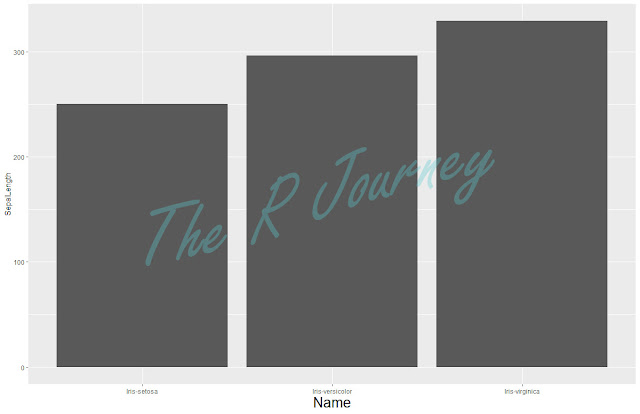
- Change font color
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue"))
Output
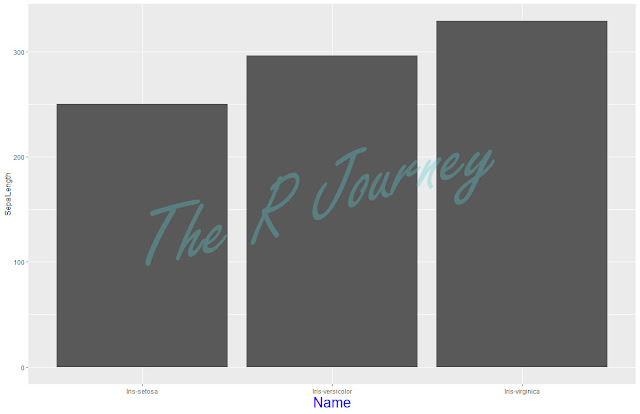
- Change font face
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic"))
Output
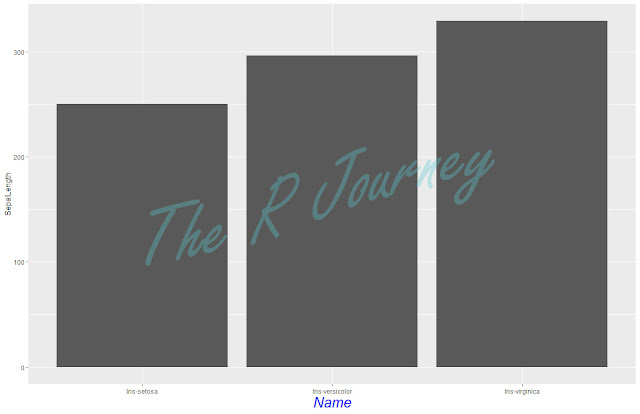
Modify the axis text.
The element modified here is axis.text.x
Note the difference between axis.title.x and axis.text.x
Change the font size, color and face
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold"))
Output
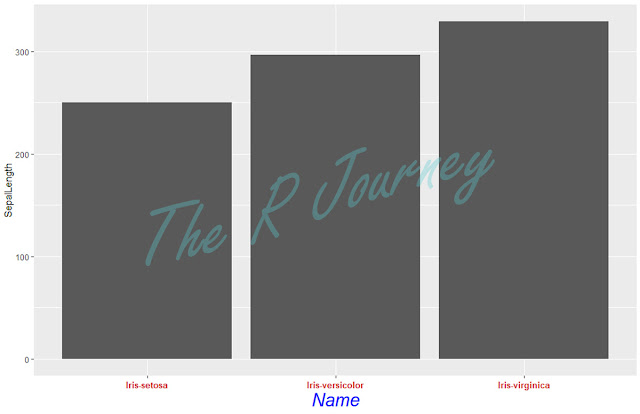
- Change the angle
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold", angle = 90, hjust = 0.5))
hjust = 0.5 to keep the text at the center of the bar.
Output
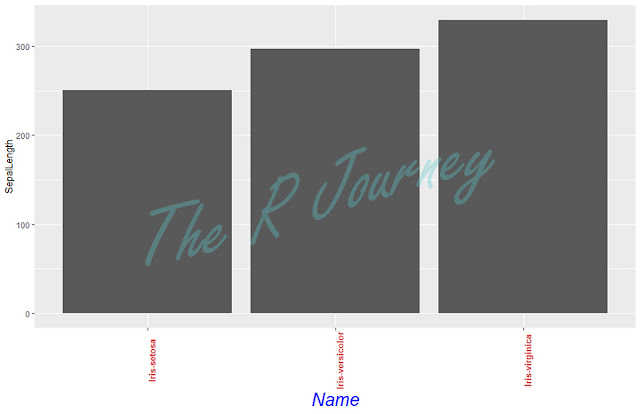
- Wrap the text
Other option is to wrap the text. For this we need library stringr. Using the function str_wrap to do this wrapping.
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold")) +
scale_x_discrete(labels = function(x) str_wrap(x, width = 10))
Output
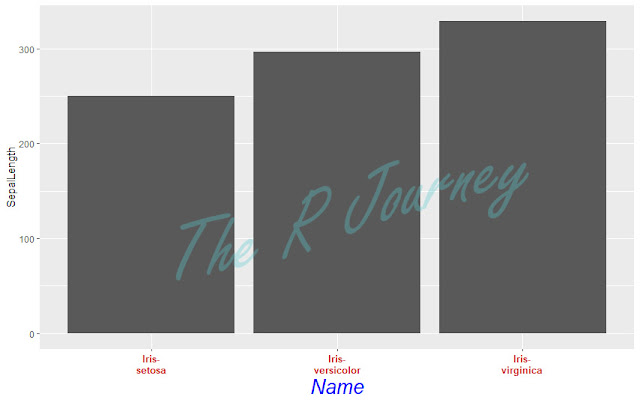
All the actions that has been explained above can be done on for axis y. But there is no axis text.
Code
ggplot(tbl, aes(x=Name, y=SepalLength, fill = Name)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold")) +
scale_x_discrete(labels = function(x) str_wrap(x, width = 10)) +
theme(axis.title.y = element_text(size = 20, color = "blue", face = "italic"))
Output
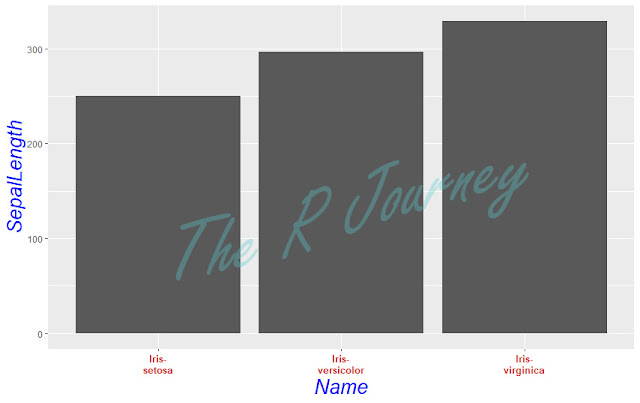
Modify the scale of y axis
It is possible to manually change the scale.
Code
ggplot(tbl, aes(x=Name, y=SepalLength)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold")) +
scale_x_discrete(labels = function(x) str_wrap(x, width = 10)) +
theme(axis.title.y = element_text(size = 20, color = "blue", face = "italic")) +
scale_y_continuous(limits = c(0,350), breaks = seq(0,350,50))
Output
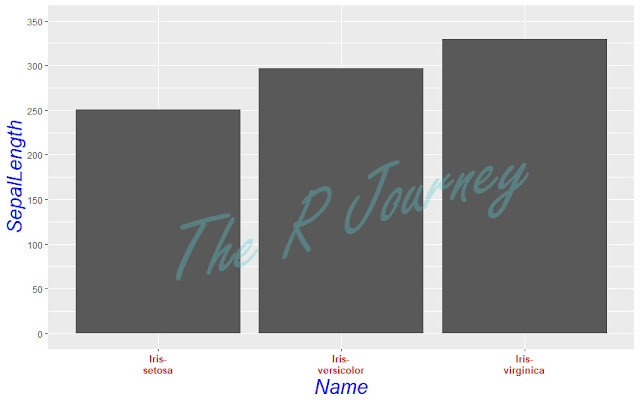
- Modify the bars
- Change the colors
By adding “fill = Name” in aes you can change the colors
Code
ggplot(tbl, aes(x=Name, y=SepalLength, fill = Name)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold")) +
scale_x_discrete(labels = function(x) str_wrap(x, width = 10))
Output
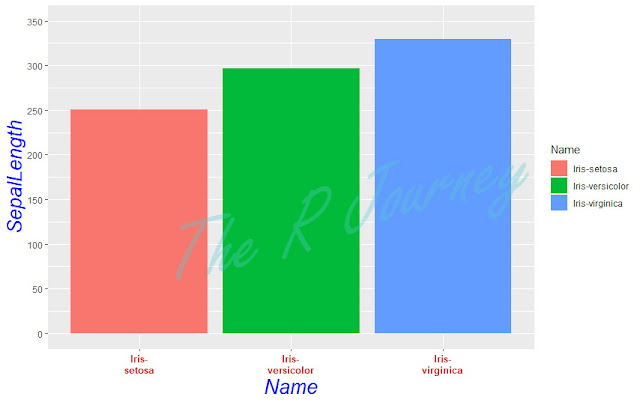
The colors are pre-defined, if you want to change the colors as per your choice the need to do a manual override.
To do that you need to now all the categories in x.
Easily done using unique function
unique(tbl$Name)
[1] "Iris-setosa" "Iris-versicolor" "Iris-virginica"
Code
ggplot(tbl, aes(x=Name, y=SepalLength, fill = Name)) +
geom_bar(stat="identity") +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold")) +
scale_x_discrete(labels = function(x) str_wrap(x, width = 10)) +
theme(axis.title.y = element_text(size = 20, color = "blue", face = "italic")) +
scale_fill_manual("legend", values = c("Iris-setosa" = "indianred3", "Iris-versicolor" = "lightcyan2", "Iris-virginica" = "darkolivegreen2"))
Output
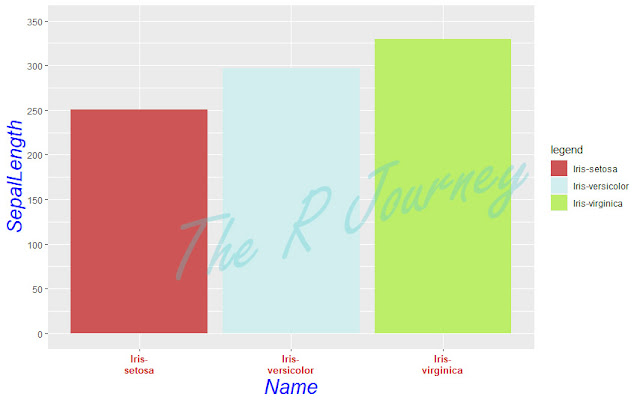
Change the width of the bars It is easily done using width = in Geom_bar
Code
ggplot(tbl, aes(x=Name, y=SepalLength, fill = Name)) +
geom_bar(stat="identity", width = 0.3) +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold")) +
scale_x_discrete(labels = function(x) str_wrap(x, width = 10)) +
theme(axis.title.y = element_text(size = 20, color = "blue", face = "italic")) +
scale_fill_manual("legend", values = c("Iris-setosa" = "indianred3", "Iris-versicolor" = "lightcyan2", "Iris-virginica" = "darkolivegreen2"))
Output
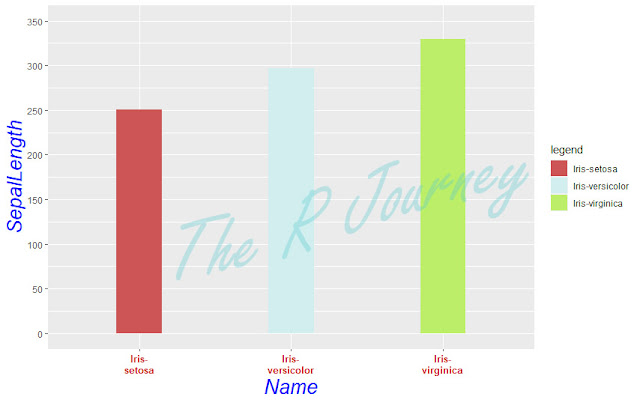
In the next post I will be discussing further configuration/modification/enhancement you can do with ggplot2.
Update I:
The y axis 0 is not starting at x axis. There is a gap.
Code
ggplot(tbl, aes(x=Name, y=SepalLength, fill = Name)) +
geom_bar(stat="identity", width = 0.3) +
theme(axis.title.x = element_text(size = 20, color = "blue", face = "italic")) +
theme(axis.text.x = element_text(size = 10, color = "firebrick3", face = "bold")) +
scale_x_discrete(labels = function(x) str_wrap(x, width = 10)) +
theme(axis.title.y = element_text(size = 20, color = "blue", face = "italic")) +
scale_y_continuous(expand = c(0, 0), limits = c(0,350), breaks = seq(0,350,50)) +
scale_fill_manual("legend", values = c("Iris-setosa" = "indianred3", "Iris-versicolor" = "lightcyan2", "Iris-virginica" = "darkolivegreen2"))
Output
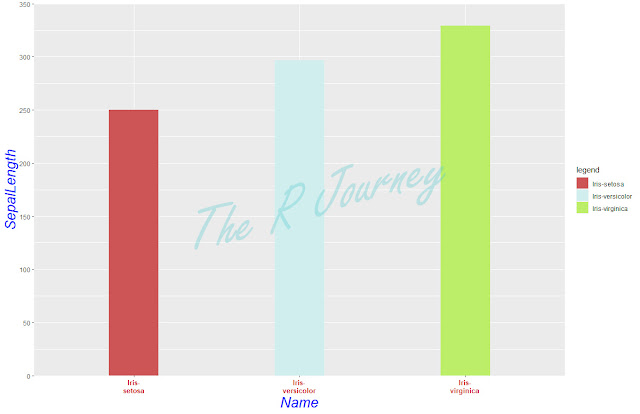
No comments:
Post a Comment